When you write an SQL query, the database engine executes the clauses in a specific order, which is different from the sequence in which you write them.
Understanding this order is crucial for optimizing queries and debugging issues efficiently. Hereโs a concise guide to the SQL query execution order:
1. ๐๐ฅ๐ข๐ ๐ฎ๐ป๐ฑ ๐๐ข๐๐ก
Purpose: Identify and join tables.
FROM: Specifies the tables to be queried.
JOIN: Combines records from related tables.
Example:
“FROM Employees
JOIN Departments ON Employees.DeptID = Departments.DeptID
“
2. ๐ช๐๐๐ฅ๐
Purpose: Filter rows based on conditions.
Filters individual rows that meet the specified criteria.
Example:
“WHERE Employees.Salary > 50000
“
3. ๐๐ฅ๐ข๐จ๐ฃ ๐๐ฌ
Purpose: Group rows based on column values.
Creates summary rows using aggregate functions.
Example:
“GROUP BY Departments.DeptName
“
4. ๐๐๐ฉ๐๐ก๐
Purpose: Filter groups based on conditions.
Filters groups created by GROUP BY.
Example:
“HAVING COUNT(Employees.EmployeeID) > 5
“
5. ๐ฆ๐๐๐๐๐ง
Purpose: Select columns for the final result.
Specifies which columns to display.
Example:
“SELECT Departments.DeptName, COUNT(Employees.EmployeeID) AS NumEmployees
“
6. ๐๐๐ฆ๐ง๐๐ก๐๐ง
Purpose: Remove duplicate rows.
Ensures uniqueness in the result set.
Example:
“SELECT DISTINCT Employees.JobTitle
“
7. ๐ข๐ฅ๐๐๐ฅ ๐๐ฌ
Purpose: Sort the result set.
Orders the rows based on specified columns.
Example:
“ORDER BY NumEmployees DESC
“
8. ๐๐๐ ๐๐ง / ๐ข๐๐๐ฆ๐๐ง
Purpose: Limit the number of rows returned.
Constrains the result set.
Example:
“LIMIT 10 OFFSET 20
“
Complete Example Query
Hereโs how it looks when put together:
“SELECT Departments.DeptName, COUNT(Employees.EmployeeID) AS NumEmployees
FROM Employees
JOIN Departments ON Employees.DeptID = Departments.DeptID
WHERE Employees.Salary > 50000
GROUP BY Departments.DeptName
HAVING COUNT(Employees.EmployeeID) > 5
ORDER BY NumEmployees DESC
LIMIT 10 OFFSET 20
“
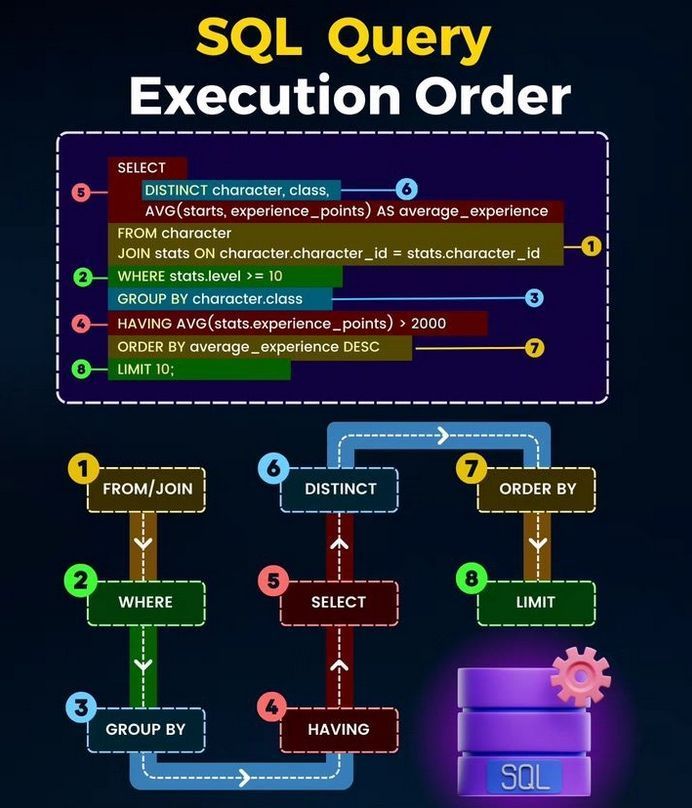